To access the Unloc API, you need to obtain a token with your API Client Credentials. The returned token is scoped to a specific resource and should be passed as a JWT Bearer in subsequent requests when working on this resource.
Different endpoints requires differently scoped tokens. The two main scopes are organization.admin
andproject.admin
, which respectively gives you access to an Organization or a Project.
If you don't know what Organizations and Projects you have permissions to, use the Resource discovery endpoint to retrieve the list of your permissions.
Learn more about how to go about it in this guide:
Authentication flow
The following diagram describes how you can get a token to fetch Locks from a Project.
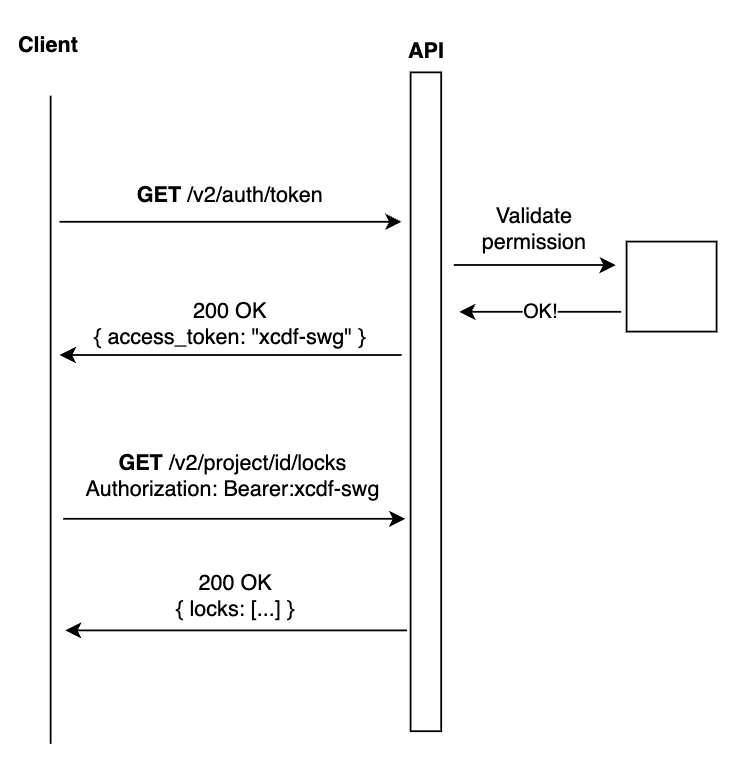
Getting the Access Token
In order to get an Access Token, make a POST request to the token
end point:
https://api.unloc.app/v2/auth/token/
For all Token requests the following parameters are required within the request's body:
- grant_type: Only
client_credentials
is supported. - client_id: Your Client ID.
- client_secret: Your Client Secret.
- scope: The required scope
In addition you need to provider either organization_id
or project_id
depending on the scope you required.
Getting a Organization Admin token
Here's and example of an Access Token request with the organization.admin
scope:
curl --request POST '<<api_url>>/v2/auth/token/' \
--header 'Content-Type: application/json' \
--data '{
"grant_type": "client_credentials",
"client_id": "**Your Client ID**",
"client_secret": "**Your Client Secret**",
"scope": "organization.admin",
"organization_id": "** Your organization id**"
}'
The response will have the following format:
{
"access_token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibm", // shortened for simplicity. Your actual token will be longer
"token_type": "bearer",
"expires_in": 3600,
"scope": [
"organization.admin"
],
"organization_id": "** The id of the Organization**",
}
Getting a Project Admin token
Here's and example of an Access Token request with the project.admin
scope:
curl --request POST '<<api_url>>/v2/auth/token/' \
--header 'Content-Type: application/json' \
--data '{
"grant_type": "client_credentials",
"client_id": "**Your Client ID**",
"client_secret": "**Your Client Secret**",
"scope": "project.admin",
"project_id": ** Your project id**
}'
The response will have the following format:
{
"access_token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibm", // shortened for simplicity. Your actual token will be longer
"token_type": "bearer",
"expires_in": 3600,
"scope": [
"project.admin"
],
"organization_id": "** The id of the Organization this Project belongs to**",
"project_id: "** The id of the Project **",
}
Did you know?
You can avoid making unnecessary extra requests to the Token request endpoint by storing your
access_token
in memory to authenticate your requests.
Using the Access Token
Unloc's API endpoints will validate for the presence of an Authorization header containing the value Bearer
followed by a space
and then the access_token
received in the Token request. Here's an example:
Authorization: Bearer mV4cI6MTY1NDAzMjI3N3IiXX0m2hU91eGGWP.muTmF5eUiOiJSb2N0c0bkwVwnwcetb
Use this header when making requests to the Unloc API.
Scopes
Organization Admin Scope
The Organization Admin Scope: organization.admin
allows you to interact with endpoints on the top level. You can list out all your Projects, and create new Projects.
Project Admin Scope
The Project Admin Scope: project.admin
provides access to Unloc's Project entities: Lock, Managed User, Key, Sharing Right and more.
Resource Discovery
Use the Resource Discovery endpoint to retrieve what permissions you have on the Client Credentials. Your client credentials can also be granted access to resources outside your own organization. To figure out what Projects and Organizations you have access to, and at what level, you can use the Resources discovery endpoint.
The endpoint returns a list of what resources you can access through the API:
{
"resources": {
"projects": [
{
"projectId": "26562a9a-a6b0-44da-956e-24b07fcb6805",
"scope": "project.admin"
},
{
"projectId": "4010c562-7f2e-43d6-935a-5d8402bb3646",
"scope": "project.installer"
}
],
"organizations": [
{
"organizationId": "b7494443-583b-497e-af46-75a2e2d571e0",
"scope": "organization.admin"
}
],
"subprojects": []
}
}